How Developers Can Enhance Their Debugging Competencies By Gustavo Woltmann
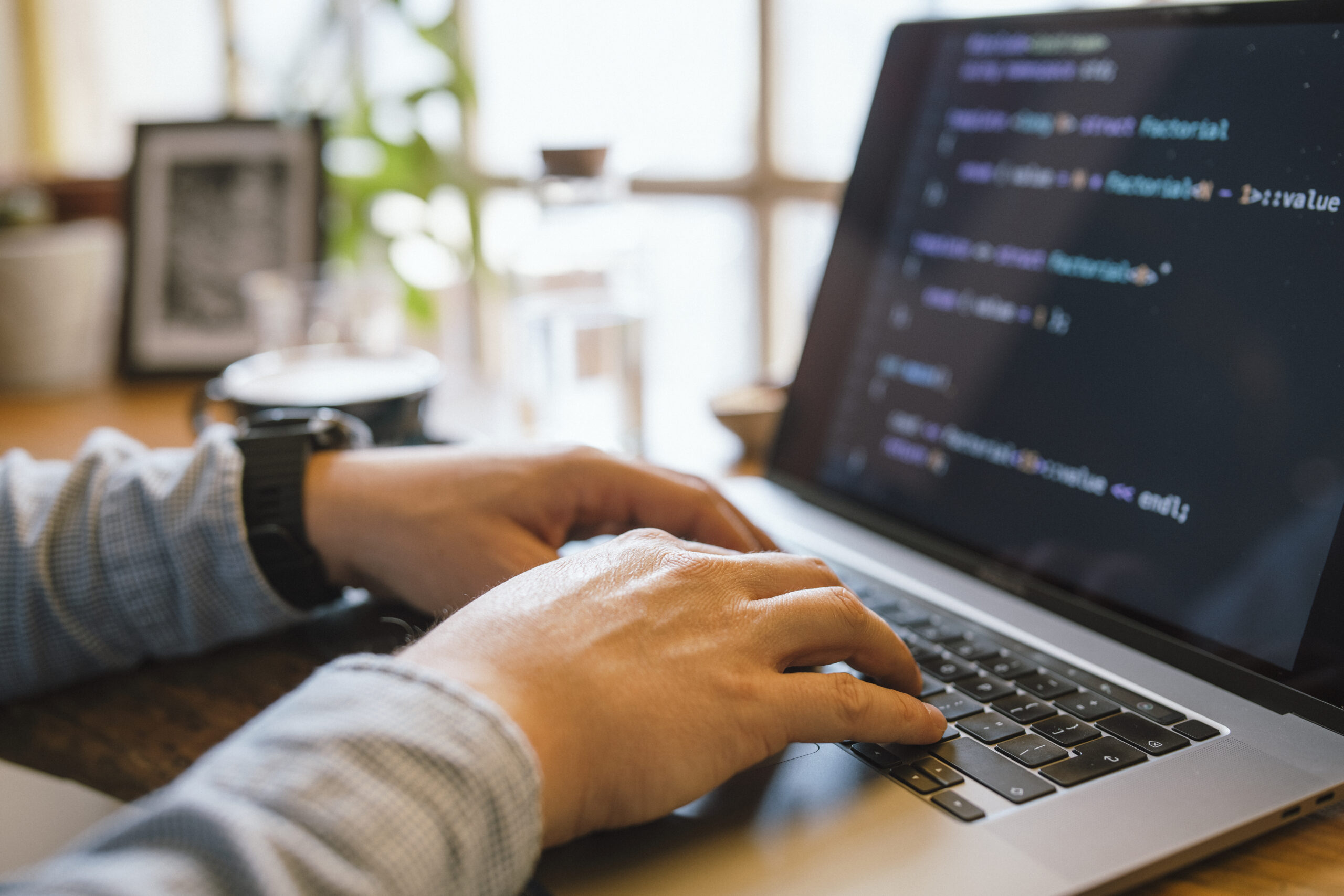
Debugging is One of the more vital — nonetheless normally ignored — expertise in a very developer’s toolkit. It isn't really pretty much correcting broken code; it’s about comprehension how and why points go Completely wrong, and learning to Believe methodically to solve troubles successfully. Irrespective of whether you are a starter or simply a seasoned developer, sharpening your debugging skills can conserve hours of disappointment and drastically boost your productiveness. Listed below are various approaches to help you developers degree up their debugging sport by me, Gustavo Woltmann.
Learn Your Resources
Among the fastest means builders can elevate their debugging capabilities is by mastering the resources they use every single day. Even though creating code is 1 part of enhancement, figuring out the way to communicate with it efficiently throughout execution is Similarly critical. Modern day development environments come Geared up with strong debugging capabilities — but lots of developers only scratch the surface of what these tools can perform.
Get, for instance, an Built-in Growth Surroundings (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These equipment enable you to set breakpoints, inspect the value of variables at runtime, action by means of code line by line, as well as modify code within the fly. When used the right way, they Permit you to notice particularly how your code behaves through execution, which is a must have for tracking down elusive bugs.
Browser developer applications, for instance Chrome DevTools, are indispensable for entrance-finish developers. They let you inspect the DOM, monitor community requests, watch authentic-time general performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and community tabs can switch aggravating UI problems into manageable jobs.
For backend or technique-stage developers, applications like GDB (GNU Debugger), Valgrind, or LLDB offer you deep control more than jogging processes and memory administration. Learning these equipment can have a steeper Studying curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, turn out to be relaxed with Model control devices like Git to know code historical past, come across the exact moment bugs had been launched, and isolate problematic modifications.
Eventually, mastering your instruments means going past default options and shortcuts — it’s about establishing an intimate knowledge of your advancement setting making sure that when challenges crop up, you’re not misplaced at nighttime. The higher you understand your equipment, the more time it is possible to commit resolving the particular challenge rather then fumbling as a result of the procedure.
Reproduce the situation
One of the more crucial — and often ignored — steps in effective debugging is reproducing the problem. Before leaping into the code or making guesses, builders will need to make a constant environment or state of affairs where the bug reliably seems. With no reproducibility, repairing a bug gets to be a activity of probability, typically bringing about wasted time and fragile code adjustments.
The first step in reproducing a problem is collecting as much context as feasible. Ask issues like: What actions triggered The problem? Which environment was it in — progress, staging, or creation? Are there any logs, screenshots, or error messages? The greater depth you have got, the less complicated it gets to be to isolate the precise circumstances below which the bug takes place.
After you’ve gathered adequate information and facts, try and recreate the problem in your neighborhood surroundings. This may imply inputting a similar info, simulating identical user interactions, or mimicking process states. If The problem seems intermittently, take into account crafting automated assessments that replicate the edge circumstances or state transitions included. These tests not merely assistance expose the issue and also prevent regressions Sooner or later.
Sometimes, The problem can be atmosphere-distinct — it'd happen only on specific functioning methods, browsers, or beneath unique configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating these kinds of bugs.
Reproducing the condition isn’t simply a step — it’s a attitude. It involves tolerance, observation, as well as a methodical technique. But when you finally can continuously recreate the bug, you might be already halfway to fixing it. By using a reproducible circumstance, You may use your debugging applications extra effectively, test potential fixes safely, and converse additional Evidently with all your workforce or buyers. It turns an summary criticism right into a concrete obstacle — Which’s wherever builders thrive.
Go through and Realize the Error Messages
Mistake messages are frequently the most precious clues a developer has when some thing goes Incorrect. Rather than looking at them as disheartening interruptions, builders really should study to deal with error messages as immediate communications through the program. They frequently tell you precisely what happened, wherever it occurred, and occasionally even why it transpired — if you know the way to interpret them.
Commence by studying the message diligently As well as in complete. Many builders, especially when less than time strain, glance at the first line and promptly commence making assumptions. But further within the mistake stack or logs could lie the correct root cause. Don’t just duplicate and paste mistake messages into search engines like google and yahoo — go through and understand them 1st.
Break the mistake down into elements. Can it be a syntax error, a runtime exception, or simply a logic error? Will it point to a certain file and line amount? What module or perform brought on it? These concerns can guideline your investigation and level you towards the responsible code.
It’s also handy to know the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically abide by predictable designs, and Mastering to recognize these can dramatically quicken your debugging approach.
Some errors are vague or generic, As well as in those situations, it’s very important to examine the context through which the error transpired. Test associated log entries, input values, and up to date variations within the codebase.
Don’t forget about compiler or linter warnings either. These usually precede much larger issues and provide hints about prospective bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Studying to interpret them the right way turns chaos into clarity, assisting you pinpoint concerns speedier, cut down debugging time, and turn into a more effective and assured developer.
Use Logging Correctly
Logging is One of the more powerful tools within a developer’s debugging toolkit. When utilised proficiently, it offers real-time insights into how an application behaves, aiding you recognize what’s occurring beneath the hood with no need to pause execution or stage with the code line by line.
An excellent logging method begins with being aware of what to log and at what stage. Widespread logging amounts contain DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for thorough diagnostic information all through enhancement, Details for standard functions (like productive begin-ups), Alert for probable challenges that don’t split the appliance, ERROR for precise challenges, and Deadly when the procedure can’t continue on.
Prevent flooding your logs with abnormal or irrelevant information. Too much logging can obscure vital messages and slow down your method. Deal with critical activities, state variations, enter/output values, and critical final decision factors in your code.
Structure your log messages website clearly and continuously. Include things like context, including timestamps, ask for IDs, and function names, so it’s much easier to trace troubles in dispersed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting the program. They’re Primarily useful in output environments in which stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about harmony and clarity. Which has a effectively-thought-out logging strategy, you could reduce the time it requires to identify issues, obtain further visibility into your purposes, and Enhance the Over-all maintainability and reliability of one's code.
Consider Similar to a Detective
Debugging is not just a technical activity—it is a sort of investigation. To efficiently establish and fix bugs, developers should strategy the method similar to a detective resolving a secret. This mindset will help stop working elaborate issues into manageable components and stick to clues logically to uncover the basis lead to.
Start out by accumulating evidence. Look at the signs and symptoms of the trouble: error messages, incorrect output, or efficiency troubles. The same as a detective surveys a criminal offense scene, accumulate just as much applicable information and facts as you can without leaping to conclusions. Use logs, exam conditions, and person stories to piece jointly a transparent image of what’s occurring.
Upcoming, sort hypotheses. Question by yourself: What may be leading to this behavior? Have any changes recently been built towards the codebase? Has this issue happened in advance of beneath equivalent circumstances? The intention should be to slim down prospects and discover prospective culprits.
Then, test your theories systematically. Seek to recreate the situation within a controlled natural environment. In case you suspect a particular functionality or part, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, request your code questions and Permit the outcomes guide you closer to the reality.
Shell out close attention to smaller specifics. Bugs often cover in the minimum expected destinations—like a lacking semicolon, an off-by-1 error, or possibly a race situation. Be extensive and affected person, resisting the urge to patch The difficulty with no thoroughly knowing it. Non permanent fixes could disguise the true problem, only for it to resurface afterwards.
Finally, retain notes on Everything you tried out and discovered. Just as detectives log their investigations, documenting your debugging system can conserve time for long term difficulties and assist Some others understand your reasoning.
By pondering similar to a detective, builders can sharpen their analytical abilities, tactic problems methodically, and grow to be simpler at uncovering concealed issues in intricate devices.
Write Exams
Composing assessments is among the simplest methods to boost your debugging skills and General advancement effectiveness. Assessments not simply assistance capture bugs early but also serve as a safety net that gives you self esteem when building variations to your codebase. A well-tested application is easier to debug because it enables you to pinpoint precisely in which and when an issue happens.
Begin with unit exams, which give attention to personal functions or modules. These little, isolated tests can rapidly reveal whether a specific piece of logic is working as expected. Any time a take a look at fails, you promptly know the place to seem, substantially lowering the time used debugging. Device checks are In particular valuable for catching regression bugs—concerns that reappear following previously remaining fastened.
Up coming, integrate integration checks and conclusion-to-stop tests into your workflow. These assist ensure that many areas of your application do the job collectively easily. They’re significantly handy for catching bugs that take place in complex devices with several elements or products and services interacting. If a thing breaks, your tests can show you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to definitely Believe critically regarding your code. To test a feature appropriately, you'll need to be aware of its inputs, anticipated outputs, and edge scenarios. This degree of knowledge Normally sales opportunities to better code framework and much less bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a powerful initial step. As soon as the check fails continually, you can target correcting the bug and watch your examination go when the issue is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In short, creating assessments turns debugging from the frustrating guessing sport into a structured and predictable course of action—helping you catch additional bugs, a lot quicker and much more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to become immersed in the issue—looking at your display for hrs, hoping Alternative right after Remedy. But The most underrated debugging instruments is actually stepping absent. Getting breaks can help you reset your intellect, cut down irritation, and infrequently see The difficulty from the new standpoint.
When you're too close to the code for too long, cognitive fatigue sets in. You might start overlooking obvious faults or misreading code that you choose to wrote just several hours before. With this condition, your brain gets considerably less productive at difficulty-solving. A brief wander, a espresso break, or perhaps switching to a different endeavor for ten–15 minutes can refresh your concentrate. Many developers report finding the foundation of a challenge once they've taken time to disconnect, permitting their subconscious get the job done inside the background.
Breaks also assistance protect against burnout, Specially in the course of longer debugging classes. Sitting in front of a screen, mentally caught, is not just unproductive but also draining. Stepping absent permits you to return with renewed energy in addition to a clearer frame of mind. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
For those who’re trapped, an excellent general guideline is usually to established a timer—debug actively for 45–sixty minutes, then take a five–10 minute crack. Use that time to maneuver about, extend, or do some thing unrelated to code. It may come to feel counterintuitive, especially underneath limited deadlines, nonetheless it actually contributes to a lot quicker and simpler debugging Ultimately.
In brief, getting breaks is not a sign of weak spot—it’s a sensible method. It gives your brain Place to breathe, increases your viewpoint, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is an element of resolving it.
Discover From Every single Bug
Each individual bug you come across is a lot more than simply a temporary setback—It really is a chance to mature as a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or simply a deep architectural issue, each one can educate you anything precious if you make an effort to mirror and review what went wrong.
Begin by asking oneself a number of essential issues as soon as the bug is fixed: What caused it? Why did it go unnoticed? Could it have been caught earlier with much better methods like unit testing, code critiques, or logging? The answers usually reveal blind spots inside your workflow or knowing and enable you to Construct more powerful coding routines shifting forward.
Documenting bugs can also be an excellent pattern. Continue to keep a developer journal or manage a log in which you Notice down bugs you’ve encountered, how you solved them, and Everything you realized. With time, you’ll start to see styles—recurring difficulties or widespread problems—which you can proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug together with your friends is often Specifically potent. Whether it’s via a Slack concept, a short write-up, or A fast information-sharing session, assisting Many others stay away from the exact same difficulty boosts staff efficiency and cultivates a much better Understanding culture.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as crucial elements of your enhancement journey. All things considered, some of the finest developers will not be the ones who publish perfect code, but individuals who continuously understand from their mistakes.
Ultimately, Each individual bug you resolve provides a brand new layer to the talent set. So following time you squash a bug, take a minute to replicate—you’ll come away a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities usually takes time, apply, and endurance — though the payoff is huge. It helps make you a far more economical, confident, and capable developer. The subsequent time you might be knee-deep in a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become much better at Whatever you do.